Mini Cash Counter Application program in C
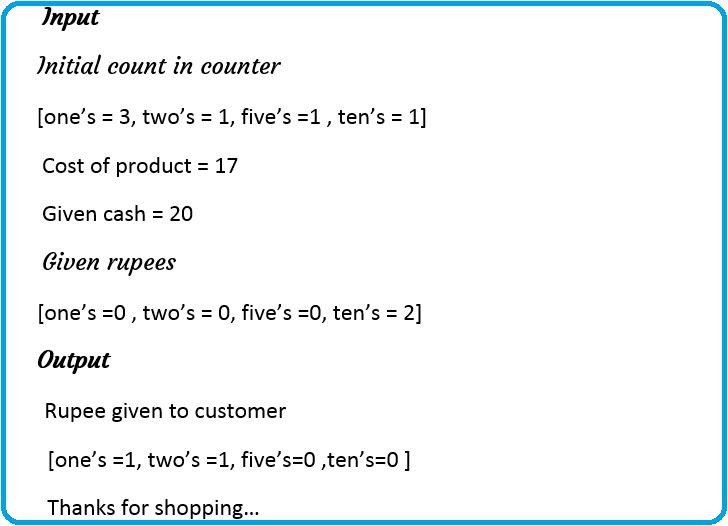
Question
Develop a simple cash counter application for all the scenarios possible during the exchange.
Logic
- Get the cash, put that into the counter(2’s or 10’s)
- Calculate the return amount in descending order, from 10’s then 5’s and so on. Because if the return amount is 5, you must give one 5 rupee not five 1 rupee right!
- Separate the given change from the cash in counter.
Algorithm
- Get the initial balance of the counter.
- Get the cost and given cash and calculate change amount.
- Get the count of each rupee given by the customer (Ex : 10’s -> 1, 5’s->1)
- Calculate the change to be given in descending order (10,5,2,1)
- Update the change amount at each step.
- Update the count of each rupee in cash counter accordingly.
Program
#include<stdio.h> int main() { /*cash -> cash given by customer change-> return amount to be given one,two,five,ten-> number of rupees ex: one =2 means two one rupee coin is in counter */ int one=0, two=0, five=0, ten=0; int one1,two1,five1,ten1,temp, i; int cash, change, cost,num; printf("enter intial count of each rupee"); scanf("%d%d%d%d",&one,&two,&five,&ten); //intial counter balance do { printf("enter cost and given cash"); scanf("%d%d",&cost,&cash); change = cash- cost; //calculate amount to return printf("enter the count of each rupee given in increasing order"); scanf("%d%d%d%d",&one1,&two1,&five1,&ten1); //count of each rupee given by customer /*adding count of rupee to the cash already present in counter*/ one+=one1; two+=two1; five+=five1; ten+=ten1; if(change==0) printf("Thank you for shopping..."); if(change<0) printf("Sorry more cash needed"); //cost exceeds the given cash /* calculating return amount in descending order from 10 to 1 Ex: generally if we need to give 3 rupee, first we give two(1) and one(1)*/ if(change>0) { if(ten!=0) { /*to find number of 10's we can give, divide by 10 ex: 25/10 = 2 => num */ num = change/10; temp = ten- num; //subtracting from number of 10's already present in counter if(temp>0) { change = change -(10*num); //Subtracting change from 10's given ex: 25-(10*2) ten = temp; printf("\nten : %d ", num); } /*if number of ten's is lesser than number of ten's to be given temp = 2-4 in such cases give all the ten's to the customer present in counter */ else{ change =change -(10*ten); printf("\nten : %d ", ten); ten= 0; //number of ten in counter is empty } } if(five!=0&&change) //change must be > 0 { num= change/5; temp = five - num; if(temp>0) { change = change-(5*num); five =temp; printf("five : %d ",num); } else { change = change -(5*five); printf("\nfive : %d ",five); five=0; } } if(two!=0&&change) { num= change/2; temp = two - num; if(temp>0) { change = change - (2*num); two = temp; printf("two : %d ",num); } else { change = change - (2*two); printf("two : %d ",two); two =0; } } if(one!=0&&change) { num = change/1; temp =one - num; if(temp>0) { change = change - num; one = temp; printf("one : %d",num); } else { change = change -(one); printf("one : %d",one); one =0; } } if(change>0) //cannot give change printf("\ninsuffient change"); else printf("\nThanks for coming"); //change =0 } printf("\nCustomers waiting in line...enter 1 if yes 0 if no: "); scanf("%d",&i); /* loop continue if more customers are waiting in line*/ }while(i); return 0; }