To print the string pattern using c
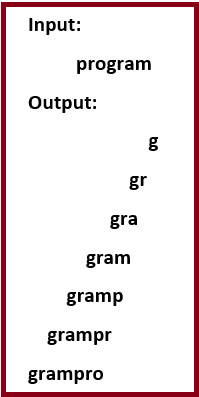
Question:
To print the string pattern using a given odd length word which should be printed from the middle of the word.
Logic:
- Get the input string from the user.
- Find the middle position of the string.
- Prints the second half of the string.
- And then prints the first half by including the second half.
Algorithm:
- Calculate the length of the input string.
- And find the middle position of the string.
- Then print the spaces according to the length.
- Print the second half of the string orderly.
- Then print the first half by including the second half that gives the pattern like structure.
Program:
#include<stdio.h> void main() { char a[20]; int i,j,n,c; clrscr(); printf("enter the string:"); gets(a); n=strlen(a);//stores the length of string c=n/2;//finds middle index of the string for(i=0;i<n;i++) { for(j=n-1;j>i;j--)//prints the spaces { printf(" "); } for(j=c;j<=c+i&&j<n;j++)//prints the right half of the string { printf("%c",a[j]); } if(i>c)//checks whether it prints all the right half elements { for(j=0;j<i-c;j++)//prints the left half of the string { printf("%c",a[j]); } } printf("\n"); } getch(); }