Implement bubble sort using recursion
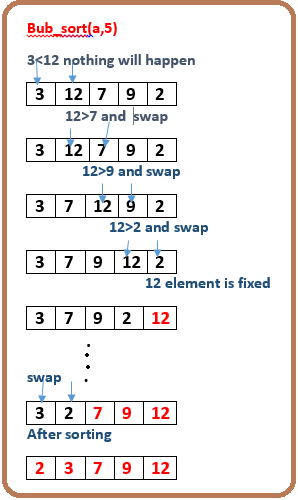
This program can implement a bubble sort algorithm using recursion.
Logic:
- Get the array elements from the user.
- Then pass a[] and length of the array to bub-sort() function.
- And check the current element with neighboring element.
- When the current element is greater then swap the elements.
- After reaching n-1th the element greater element is fixed in the last position.
- Then call the function recursively by decrementing length by 1.
- When the value of n becomes 1 then all elements are in sorted order.
- And print the sorted elements.
Program:
#include<stdio.h> void bub_sort(int a[],int n) { int i,temp; if(n>1) { for(i=0;i<n-1;i++) { if(a[i]>a[i+1]) //swaps when cur element greater than neighbouring element { temp=a[i]; a[i]=a[i+1]; a[i+1]=temp; } } bub_sort(a,n-1); //calls recursively without including last element of a[] } } void main() { int i,n,a[20]; clrscr(); printf("Enter the length of array:"); scanf("%d",&n); printf("enter the elements:"); for(i=0;i<n;i++) { scanf("%d",&a[i]); } bub_sort(a,n); //calls the bubble function for(i=0;i<n;i++) { printf("%d ",a[i]); } getch(); }
You Might also like:
Python Program To Sort Elements Using Selection Sort