Print array in Pendulum arrangement | C Program
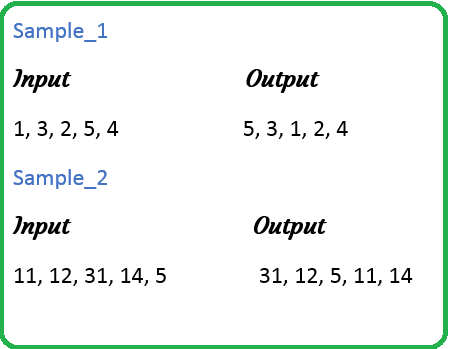
Question
Write a program to print array in pendulum arrangement. Given a list of integers arrange as
i) the minimum element out of the list of integers, must come in center position of array
ii)The number in the ascending order, next to minimum, goes to right and next higher number goes to left. It continues in this fashion.
Logic
- First, sort the array. Store the minimum element in the center position of a new array.
- Traverse the sorted array and store one element in the right side and next element in the left side of the middle position.
- Increment the right and left index and repeat the same procedure for all the elements.
- Print the new array with pendulum arrangement.
Program
#include<stdio.h> #include<stdlib.h> int main() { int r=1,l=1,i,j,n,mid,temp; printf("enter the number of elements"); scanf("%d",&n); int *arr = (int*)malloc(sizeof(int)*n); int *pen =(int*)malloc(sizeof(int)*n); if(arr == NULL || pen == NULL) { printf("no memory available"); exit(0); } printf("enter elements"); for(i=0;i<n;i++) { scanf("%d",&arr[i]); } /*Sorting the array*/ for(i=n;i>0;i--) { for(j=0;j<i-1;j++) { if(arr[j]>arr[j+1]) { temp = arr[j]; arr[j] = arr[j+1]; arr[j+1] = temp; } } } mid=n/2; pen[mid]=arr[0]; //storing the smallest in middle position for(i=1;i<n;i++) { /*If i is odd, then store in right side*/ if(i%2!=0) { pen[mid+r] = arr[i]; r++; //increment right count } /*If i is even*/ else { pen[mid-l] = arr[i]; l++; //increment left count } } /*printing pendulum array*/ for(i=0;i<n;i++) { printf("%d\n",pen[i]); } /*Unallocating the heap memory*/ free(pen); free(arr); }
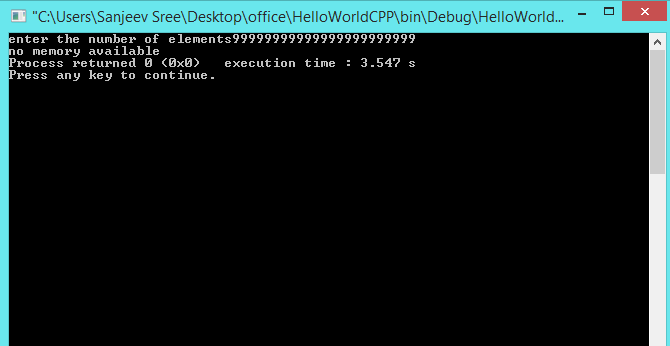
Analysis
Time complexity –O(squareof(n)) , because we sort the elements n square complexity. Next for loop is O(n). So square(n) + n => square(n)
Space complexity – O(n), because we create another array pen relative to the size of the input.
You might also like…
Implement bubble sort using recursion