No of identical digit showed by digital clock | Infosys
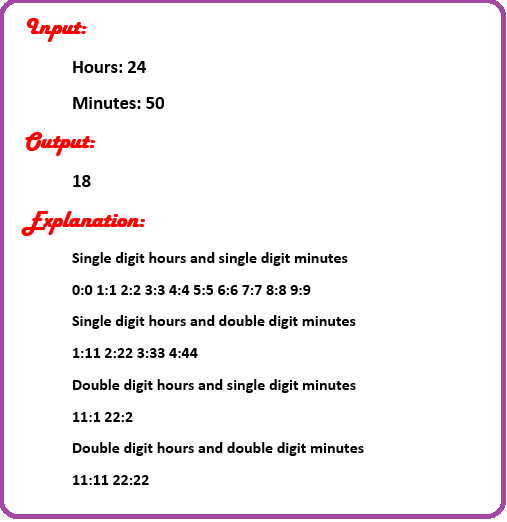
Given a digital clock, the task is to find how many times the clock shows identical time. A specific time is said to be identical if every digit in the hours and minutes is the same i.e. the time is of type T: T, T: TT, TT: T, TT: TT.
Logic:
- Get the hours and minutes from the user.
- Then count the number of single digit hours identical with single and double-digit minutes.
- And count the number of double-digit hours identical with single and double-digit minutes.
- At last print the count which is the number of occurrences of identical digits.
Program:
#include<stdio.h> void main() { int hours,minutes,count=1,i;//count is initialized to 1 because for inputs 0:0 occur printf("enter the hours:"); scanf("%d",&hours); printf("enter the minutes:"); scanf("%d",&minutes); //single digit hours for(i=1;i<=9&&i<hours;i++) { if(i<minutes)//single digit minutes count++; if(i*10+i<minutes)//double digit minutes count++; } //double digit hours for(i=11;i<=99&&i<hours;i+=11) { if(i<minutes)//double digit minutes count++; if(i%10<minutes)//single digit minutes count++; } printf("Count:%d",count); }