Program to perform string operations | ZOHO
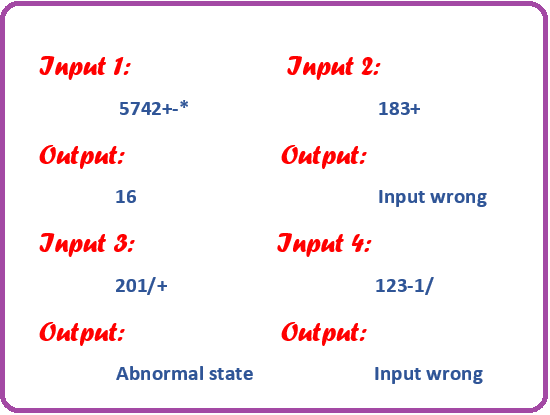
Program to perform string operations. ie Operator precedence is not considered
Logic:
- Get the input from the user which contains numbers followed by operators.
- The count of numbers is n means then operators must be n-1 otherwise input will be wrong. ie.,123-+(correct)
- The operators and numbers don’t collide they must be in a separated manner ie.,1234-+1/(wrong)
- Take the first two elements to take the first operator to perform the operation.
- The result is assigned as the first element and the second element is the next number in input.
- At last print the result of the string operations.
Explanation:
- Let us consider input 1 ie,5742+-*
- First, check the given input is correct or not by using two conditions
1. Number length is n means and operator length is n-1
2. The starting letter of the string should be number and the number and operator should be in a separated manner ie, doesn’t collide
- For the above input satisfies both the conditions where n=4 and operator length is 3
- Take the first number and the second number using ASCII where b=5 and c=7 and k=4 which is the length of the numbers, then take the operator which is in k+0th position and perform the corresponding operation
- Now b=12 and c=4 then take the operator in k+1th position and perform the subtraction operation
- Now, b=8 and c=2 and the operator found in k+2th position is * and the result is 16
- Then print the output when no abnormal state is found
Program:
#include<stdio.h> void main() { //k is count of numbers //l is count of Operators //j act as a marker check collision ocuurs or not //d act as a marker for occurance of abnormal state //b, c contains first and second element for operations char a[30]; int i,j=0,n,k=0,l=0,c,b,d=1; scanf("%s",&a); n=strlen(a); for(i=0;i<n;i++) { if(a[i]>=48&&a[i]<=58) { if(l==0) { k++; //length of number } else { j=1; //wrong input ie 123*1/+ break; } } else { l++; } } if(k!=l+1||j==1) //wrong input ie 1234*/ { printf("Input wrong"); } else { l=0; b=a[0]-48; //first element for arithmetic operation for(i=1;i<k;i++) { c=a[i]-48; //second element for arithmetic operation switch(a[k+l]) //select the operator { //b is assigned as first element for every time case '+': b=b+c; break; case '-': b=b-c; break; case '*': b=b*c; break; case '/': if(c==0) //abnormal state of 1/0 error { printf("Abnormal state"); d=0; } b=b/c; break; } if(d==0) { break; } l++; //switch to next operator } if(d!=0) //print the result { printf("%d",b); } } }