To find string length using recursion
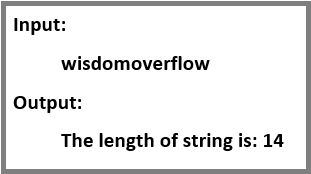
Question:
Write a program to find string length using recursion.
Logic:
- Get the input string from the user.
- Then count the characters in the string.
- String length found when reach the \0.
Algorithm:
- Get the input string from the user.
- Pass the string as an argument.
- Then count the number of characters in the string.
- The string length is found by passing string recursively.
- Then return the count value by reaching \0.
Program:
#include<stdio.h> void main() { char a[30]; clrscr(); printf("enter the string:"); gets(a); printf("the length of string is %d",len(a)); getch(); } int len(char a[]) //gets the string as an argument { static int count=0; //it initializes the i value for first time only if(a[count]!='\0') //checks every position in the string { count++; len(a); // passes the string recursively } else { return count; //returns the length of string } }