Count of each character in a string
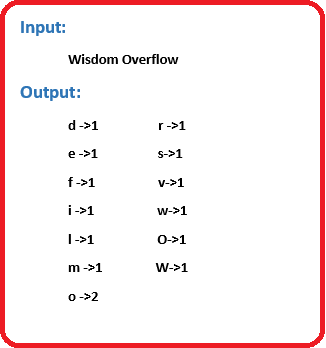
Question: Find the character count of each character in a string.
Output:
Logic:
- Get the input string from the user
- Check the small alphabets and store the value of a to z in the index 0 to 25 using ASCII of characters
- Check the capital alphabets and store the value of A to Z in the index 26 to 51 using ASCII of characters
- And print the alphabets using their corresponding index in count[] array
ASCII Code
ASCII is the acronym for the American Standard Code for Information Interchange. It is a code for representing 128 English characters as numbers, with each letter assigned a number from 0 to 127. For example, the ASCII code for uppercase A is 65.
The standard ASCII Character Set
The standard ASCII character set uses just 7 bits for each character. There are several larger character sets that use 8 bits, which gives them 128 additional characters. The extra characters are used to represent non-English characters, graphics symbols, and mathematical symbols
Difference between ASCII and HTML Code
The original ASCII code only had a range of 128 characters which is very limited in the range of characters. It basically only supports the English character set. You could have used the extended ASCII characters which ranged from 128 to 255. Because the ASCII code range is 0 to 255 it can fit inside 1 byte of data.
The HTML code is based on the different character sets that can range from a single-byte character set such as Latin-1 (ISO-8859-1) or UTF-8 which uses multiple bytes to represent a character. Using a character set such as UTF-8 gives us a much larger range of character sets.
Program:
#include<stdio.h> #include<string.h> int main() { char input[20],count[52]={0}; int index=0,alpha_index; printf("Enter the string:"); gets(input); while(input[index]!='\0') { if(input[index]>='a'&&input[index]<='z') //check for small alphabets { alpha_index=input[index]-'a'; count[alpha_index]++; //stores count of a to z in 0 to 25 } else if(input[index]>='A'&&input[index]<='Z') //check for capital alphabets { alpha_index=input[index]+26-'A'; count[alpha_index]++; //stores count of A to Z in 26 to 51 } index++; } for(index=0;index<52;index++) //prints the character count { if(count[index]!=0) { if(index<26) //prints small alphabets printf("%c->%d\n",index+'a',count[index]); else //prints capital alphabets printf("%c->%d\n",index+39,count[index]); } } return 0; }