Merge two arrays in sorted order
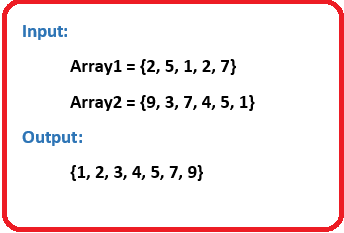
Question: Merge two arrays in sorted order without duplicate (without using extra array)
Output:
Merge Two Arrays
Method 1:
Sort both array1 and array2 separately and eliminate the duplicates. Add array1 elements into the new array and then add array2 elements into the new array in sorted order.
Method 2:
Sort both array1 and array2 separately and also elimate the duplicates. Then add array2 elements into array1 in sorted order.
Method 3:
Sort array1 and also eliminate the duplicates and then add array2 into array1 in sorted order. The below code is done in this method.
Logic:
- To merge two arrays, get two array elements from the user
- Sort the first array and also eliminate the duplicates in that array
- Insert the array2 elements into array1 by using some conditions
- Array2 element is equal to the array1 element is duplicate and we can’t that element into the array1
- Find the index of array1 where the array1 element is greater than array2 element and insert array2 element into the array1
- Add the element into array1’s last position when no element is greater then array2 with array1
- Then print the merged array
About C arrays More>>
Program:
void main() { int *array1,*array2,length1,length2,index1,index2,index3,temp_var,mark=0; printf("Enter the length of two arrays:"); scanf("%d%d",&length1,&length2); array1=(int*)malloc((length1+length2)*sizeof(int)); array2=(int*)malloc(length2*sizeof(int)); printf("Enter first array elements:"); for(index1=0;index1<length1;index1++) scanf("%d",&array1[index1]); printf("Enter second array elements:"); for(index1=0;index1<length2;index1++) scanf("%d",&array2[index1]); for(index1=0;index1<length1;index1++) //sort the first array and eliminating duplicates { for(index2=index1+1;index2<length1;index2++) { if(array1[index1]==array1[index2]) //duplicates eliminated { for(index3=index2;index3<length1;index3++) { temp_var=array1[index3]; array1[index3]=array1[index3+1]; array1[index3+1]=temp_var; } length1--; } else if(array1[index1]<array1[index2]) { temp_var=array1[index1]; array1[index1]=array1[index2]; array1[index2]=temp_var; } } } for(index2=0;index2<length2;index2++) //merge second array into first array in sorted order and duplicates are eliminated { for(index1=0;index1<length1;index1++) { if(array2[index2]==array1[index1]) //duplicates eliminated { mark=1; break; } else if(array2[index2]<array1[index1]) { mark=1; for(index3=length1;index3>index1;index3--) //move the elements one position right { array1[index3]=array1[index3-1]; } array1[index1]=array2[index2]; length1++; break; } } if(mark==0) //copy the element when no other element is greater then index2 of array2 { array1[index1]=array2[index2]; length1++; } } for(index1=0;index1<length1;index1++) { printf("%d ",array1[index1]); } }